[C#] ステータスバー(statusstrip)の見た目をカスタマイズする方法
C#のWindows Formsアプリケーションでステータスバー(StatusStrip)の見た目をカスタマイズするには、いくつかの方法があります。
まず、StatusStripのプロパティを設定して、背景色やフォントを変更できます。
BackColor
プロパティで背景色を、Font
プロパティでフォントスタイルを指定します。
また、RenderMode
プロパティをProfessional
やSystem
に設定することで、全体のスタイルを変更できます。
さらに、カスタム描画を行うために、StatusStrip
のPaint
イベントをオーバーライドし、Graphics
オブジェクトを使用して独自の描画を行うことも可能です。
これにより、より細かいデザインのカスタマイズが可能になります。
ステータスバーの見た目をカスタマイズする方法
背景色の変更
C#のWindowsフォームアプリケーションで、ステータスバーの背景色を変更するには、StatusStrip
コントロールのBackColor
プロパティを設定します。
以下のサンプルコードでは、背景色を青色に変更しています。
partial class MyForm : Form
{
private StatusStrip statusStrip;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusStrip.BackColor = Color.Blue; // 背景色を青に設定
this.Controls.Add(statusStrip);
}
}
このコードを実行すると、ステータスバーの背景色が青色に変更されます。
フォントの変更
ステータスバーのフォントを変更するには、StatusStrip
のFont
プロパティを設定します。
以下のサンプルコードでは、フォントを MS Gothic
に変更しています。
partial class MyForm : Form
{
private StatusStrip statusStrip;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusStrip.Font = new Font("MS Gothic", 12, FontStyle.Bold); // フォントをMS Gothicに設定
this.Controls.Add(statusStrip);
}
}
このコードを実行すると、ステータスバーのフォントが MS Gothic
に変更され、サイズも12ポイントの太字になります。
アイコンや画像の追加
ステータスバーにアイコンや画像を追加するには、ToolStripStatusLabel
を使用します。
以下のサンプルコードでは、アイコンを追加しています。
partial class MyForm : Form
{
private StatusStrip statusStrip;
private ToolStripStatusLabel statusLabel;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusLabel = new ToolStripStatusLabel();
statusLabel.Image = Image.FromFile("icon.png"); // アイコンを設定
statusLabel.Text = "状態: 正常"; // テキストを設定
statusStrip.Items.Add(statusLabel);
this.Controls.Add(statusStrip);
}
}
このコードを実行すると、ステータスバーに指定したアイコンとテキストが表示されます。
サイズと配置の調整
ステータスバーのサイズや配置を調整するには、StatusStrip
やToolStripStatusLabel
のプロパティを使用します。
以下のサンプルコードでは、ラベルのサイズを変更し、中央に配置しています。
partial class MyForm : Form
{
private StatusStrip statusStrip;
private ToolStripStatusLabel statusLabel;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusLabel = new ToolStripStatusLabel();
statusLabel.Size = new Size(200, 20); // サイズを設定
statusLabel.Alignment = ToolStripItemAlignment.Center; // 中央に配置
statusLabel.Text = "状態: 正常"; // テキストを設定
statusStrip.Items.Add(statusLabel);
this.Controls.Add(statusStrip);
}
}
このコードを実行すると、サイズが200×20ピクセルのラベルが中央に配置されたステータスバーが表示されます。
高度なカスタマイズ
RenderModeの設定
StatusStrip
のRenderMode
プロパティを使用すると、ステータスバーの描画スタイルを変更できます。
これにより、アプリケーションのテーマに合わせた外観を実現できます。
以下のサンプルコードでは、RenderMode
をProfessional
に設定しています。
partial class MyForm : Form
{
private StatusStrip statusStrip;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusStrip.RenderMode = ToolStripRenderMode.Professional; // 描画スタイルをProfessionalに設定
this.Controls.Add(statusStrip);
}
}
このコードを実行すると、ステータスバーがプロフェッショナルなスタイルで描画されます。
RenderMode
には、System
やProfessional
、ManagerRenderMode
などのオプションがあります。
カスタム描画の実装
カスタム描画を実装することで、ステータスバーの外観をさらに自由にカスタマイズできます。
StatusStrip
のPaint
イベントを利用して、独自の描画処理を行います。
以下のサンプルコードでは、カスタム背景を描画しています。
partial class MyForm : Form
{
private StatusStrip statusStrip;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusStrip.Paint += StatusStrip_Paint; // Paintイベントにハンドラを追加
this.Controls.Add(statusStrip);
}
private void StatusStrip_Paint(object sender, PaintEventArgs e)
{
// カスタム背景を描画
e.Graphics.FillRectangle(Brushes.LightGreen, e.ClipRectangle); // 背景を緑色に設定
}
}
このコードを実行すると、ステータスバーの背景がカスタム描画によって緑色に変更されます。
スタイルの統一とテーマの適用
アプリケーション全体のスタイルを統一するために、StatusStrip
の外観を他のコントロールと一致させることが重要です。
以下のサンプルコードでは、アプリケーション全体にテーマを適用する方法を示します。
partial class MyForm : Form
{
private StatusStrip statusStrip;
public MyForm()
{
InitializeComponent();
// テーマの設定
this.BackColor = Color.White; // フォームの背景色を白に設定
this.Font = new Font("Arial", 10); // フォーム全体のフォントをArialに設定
statusStrip = new StatusStrip();
statusStrip.BackColor = Color.LightGray; // ステータスバーの背景色を薄灰色に設定
statusStrip.Font = this.Font; // フォームのフォントをステータスバーに適用
this.Controls.Add(statusStrip);
}
}
このコードを実行すると、フォーム全体のスタイルが統一され、ステータスバーも同様のテーマが適用されます。
これにより、ユーザーに一貫した視覚体験を提供できます。
ステータスバーの動的な更新
プログラムによるテキストの変更
ステータスバーのテキストをプログラムから動的に変更するには、ToolStripStatusLabel
のText
プロパティを使用します。
以下のサンプルコードでは、ボタンをクリックすることでステータスバーのテキストを変更しています。
partial class MyForm : Form
{
private StatusStrip statusStrip;
private ToolStripStatusLabel statusLabel;
private Button changeTextButton;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusLabel = new ToolStripStatusLabel();
statusLabel.Text = "初期状態"; // 初期テキストを設定
statusStrip.Items.Add(statusLabel);
this.Controls.Add(statusStrip);
changeTextButton = new Button();
changeTextButton.Text = "テキストを変更";
changeTextButton.Click += ChangeTextButton_Click; // ボタンクリックイベントにハンドラを追加
this.Controls.Add(changeTextButton);
}
private void ChangeTextButton_Click(object sender, EventArgs e)
{
statusLabel.Text = "状態: 更新されました"; // テキストを変更
}
}
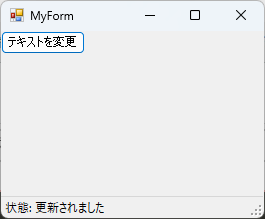
このコードを実行し、ボタンをクリックすると、ステータスバーのテキストが「状態: 更新されました」に変更されます。
イベントに応じた表示内容の更新
特定のイベントに応じてステータスバーの内容を更新することも可能です。
以下のサンプルコードでは、フォームのサイズが変更されたときに、ステータスバーに新しいサイズを表示します。
partial class MyForm : Form
{
private StatusStrip statusStrip;
private ToolStripStatusLabel statusLabel;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusLabel = new ToolStripStatusLabel();
statusStrip.Items.Add(statusLabel);
this.Controls.Add(statusStrip);
this.SizeChanged += MyForm_SizeChanged; // サイズ変更イベントにハンドラを追加
}
private void MyForm_SizeChanged(object sender, EventArgs e)
{
statusLabel.Text = $"サイズ: {this.ClientSize.Width} x {this.ClientSize.Height}"; // 新しいサイズを表示
}
}
このコードを実行し、フォームのサイズを変更すると、ステータスバーに新しいサイズが表示されます。
タイマーを使った自動更新
Timer
を使用して、定期的にステータスバーの内容を自動的に更新することもできます。
以下のサンプルコードでは、1秒ごとに現在の時刻を表示します。
partial class MyForm : Form
{
private StatusStrip statusStrip;
private ToolStripStatusLabel statusLabel;
private Timer timer;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusLabel = new ToolStripStatusLabel();
statusStrip.Items.Add(statusLabel);
this.Controls.Add(statusStrip);
timer = new Timer();
timer.Interval = 1000; // 1秒ごとに更新
timer.Tick += Timer_Tick; // タイマーのTickイベントにハンドラを追加
timer.Start(); // タイマーを開始
}
private void Timer_Tick(object sender, EventArgs e)
{
statusLabel.Text = $"現在の時刻: {DateTime.Now.ToString("HH:mm:ss")}"; // 現在の時刻を表示
}
}
このコードを実行すると、ステータスバーに1秒ごとに現在の時刻が更新されて表示されます。
これにより、リアルタイムで情報を提供することができます。
応用例
マルチステータスバーの実装
複数のステータスバーを使用することで、異なる情報を同時に表示することができます。
以下のサンプルコードでは、2つのStatusStrip
を作成し、それぞれ異なる情報を表示しています。
partial class MyForm : Form
{
private StatusStrip statusStrip1;
private StatusStrip statusStrip2;
private ToolStripStatusLabel statusLabel1;
private ToolStripStatusLabel statusLabel2;
public MyForm()
{
InitializeComponent();
// 最初のステータスバー
statusStrip1 = new StatusStrip();
statusLabel1 = new ToolStripStatusLabel("情報: 正常");
statusStrip1.Items.Add(statusLabel1);
this.Controls.Add(statusStrip1);
// 2つ目のステータスバー
statusStrip2 = new StatusStrip();
statusLabel2 = new ToolStripStatusLabel("警告: 注意が必要");
statusStrip2.Items.Add(statusLabel2);
this.Controls.Add(statusStrip2);
// 位置を調整
statusStrip1.Dock = DockStyle.Top; // 上部に配置
statusStrip2.Dock = DockStyle.Bottom; // 下部に配置
}
}
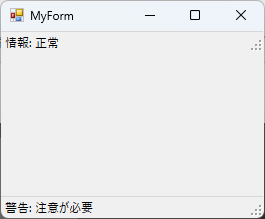
このコードを実行すると、上部に正常な情報を表示するステータスバーと、下部に警告を表示するステータスバーが表示されます。
ステータスバーを使った進捗表示
ステータスバーを使用して、処理の進捗状況を表示することができます。
以下のサンプルコードでは、ProgressBar
を使用して進捗を表示しています。
partial class MyForm : Form
{
private StatusStrip statusStrip;
private ToolStripProgressBar progressBar;
private Button startButton;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
progressBar = new ToolStripProgressBar();
statusStrip.Items.Add(progressBar);
this.Controls.Add(statusStrip);
startButton = new Button();
startButton.Text = "処理開始";
startButton.Click += StartButton_Click; // ボタンクリックイベントにハンドラを追加
this.Controls.Add(startButton);
}
private async void StartButton_Click(object sender, EventArgs e)
{
progressBar.Value = 0; // 初期値を設定
progressBar.Maximum = 100; // 最大値を設定
for (int i = 0; i <= 100; i++)
{
progressBar.Value = i; // 進捗を更新
await Task.Delay(50); // 処理の遅延をシミュレート
}
}
}
このコードを実行し、「処理開始」ボタンをクリックすると、ステータスバーに進捗が表示されます。
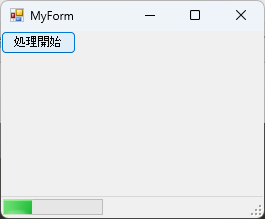
進捗バーが100%に達するまで、進捗状況が更新されます。
ステータスバーを使ったユーザー通知
ステータスバーを利用して、ユーザーに通知メッセージを表示することもできます。
以下のサンプルコードでは、ボタンをクリックすることで通知メッセージを表示し、数秒後に自動的に消去します。
partial class MyForm : Form
{
private StatusStrip statusStrip;
private ToolStripStatusLabel statusLabel;
private Button notifyButton;
public MyForm()
{
InitializeComponent();
statusStrip = new StatusStrip();
statusLabel = new ToolStripStatusLabel();
statusStrip.Items.Add(statusLabel);
this.Controls.Add(statusStrip);
notifyButton = new Button();
notifyButton.Text = "通知を表示";
notifyButton.Click += NotifyButton_Click; // ボタンクリックイベントにハンドラを追加
this.Controls.Add(notifyButton);
}
private async void NotifyButton_Click(object sender, EventArgs e)
{
statusLabel.Text = "通知: 処理が完了しました"; // 通知メッセージを表示
await Task.Delay(3000); // 3秒待機
statusLabel.Text = ""; // メッセージを消去
}
}
このコードを実行し、「通知を表示」ボタンをクリックすると、ステータスバーに通知メッセージが表示され、3秒後に消去されます。
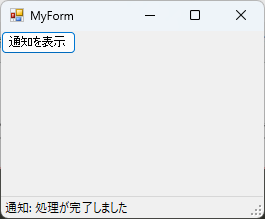
これにより、ユーザーに重要な情報を簡単に伝えることができます。
まとめ
この記事では、C#のWindowsフォームにおけるステータスバーの見た目をカスタマイズする方法や、動的な更新の実装方法について詳しく解説しました。
具体的には、背景色やフォントの変更、アイコンの追加、サイズや配置の調整、さらにはマルチステータスバーの実装や進捗表示、ユーザー通知の方法についても触れました。
これらの技術を活用することで、アプリケーションのユーザーインターフェースをより魅力的にし、ユーザーにとって使いやすいものにすることが可能です。
ぜひ、実際のプロジェクトにこれらのテクニックを取り入れて、より良いアプリケーションを作成してみてください。